Hi, I am Wendyanto, a front-end developer intern at Bukit Vista. I’m passionate about web development and love to learn new things.
This article will discuss important programming best practices. This includes DRY (Don’t Repeat Yourself), layered architecture, and factory function. These best practices can help you write more maintainable, reusable, and efficient code. As a front-end developer intern, I’m still learning about these best practices, but I’ve already found them helpful. With this article, I will share my understanding of these best practices.
DRY (Don’t Repeat Yourself)
DRY, or Don’t Repeat Yourself, is a software development principle stating that every piece of knowledge or code should have a single, unambiguous, authoritative representation within a system. In other words, you should avoid writing the same code repeatedly in your codebase.
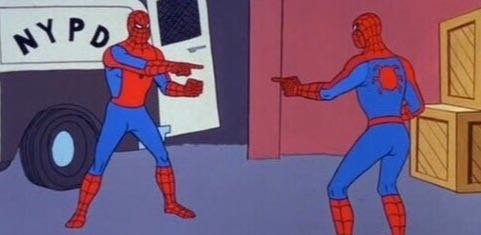
What are the benefits of following this principle?
- It makes your code more maintainable. If you need to change a piece of code, you only need to change it in one place.
- It makes your code more reusable. You can reuse code written in a DRY way in different parts of your program.
- It makes your code more efficient. DRY code is typically smaller and faster than code that repeats itself.
Here are some tips for following the DRY principle:
- Use functions and classes to encapsulate code. This makes it easier to reuse your code and to avoid repeating yourself.
- Use variables and constants to store and reference common values. This makes your code more readable and easier to maintain.
- Use data structures like arrays and objects to organize your code. This can help you to avoid repeating yourself and to make your code more efficient.
Layered Architecture
A layered architecture is a software design pattern that divides an application into multiple layers. Each layer is responsible for a specific task, and the layers are typically arranged in a hierarchy. This architectural approach helps structure and maintain complex software systems, making them more scalable, maintainable, and understandable.
There are some benefits of applying this architecture, especially on bigger-scale projects:
- Modularity: Layers are isolated, making modifying or replacing one layer easier without affecting the others. This structure supports code reusability and promotes the separation of concerns.
- Scalability: Makes your code more scalable. You can easily add new features or functionality to your application by adding or modifying existing layers.
- Maintainability: Simplifies debugging, testing, and maintenance so developers can focus on a specific layer without worrying about the other layer in the application.
Here are some tips if you want to apply layered architecture:
- Identify the different tasks that your application needs to perform.
- Group the tasks into layers, each responsible for a specific task.
- Arrange the layers in a hierarchy, with the lower layers providing services to the higher layers.
- Use interfaces to communicate between the layers. This makes your code more decoupled and easier to test.
Factory Function
A factory function is a design pattern used in software development to create and return objects or functions. It is a function that encapsulates the process of creating and initializing objects, abstracting the details of object creation from the calling code. Factory functions are often used to simplify creation, manage object instantiation, and promote encapsulation.
To make it more clear, take a look at the code example below:
function createPerson(name, age) {
const person = {};
person.name = name;
person.age = age;
person.sayHi= function() {
console.log(`Hi, I'm ${person.name}. ${person.age} y.o human.`);
};
return person;
}
const wendy = createPerson("Wendy", 99);
const yanto = createPerson("Yanto", 100);
wendy.sayHi();
yanto.sayHi();
In the example above, the factory function abstracts the process of creating personal objects with specific properties and methods. It encapsulates the details of object creation and ensures that every person’s object has the same structure.
Factory functions are a flexible and powerful design pattern used to manage object creation in a clean and organized way. They are often used in languages like JavaScript to create objects and functions that adhere to certain patterns and conventions.
Conclusion
DRY, layered architecture and factory are important programming best practices that can help you write more maintainable, reusable, and efficient code. By following these best practices, you can improve the quality of your code and make it easier to develop, maintain, and test your applications.
“That’s why programmers are slow, and slow because they make a mess” – Uncle Bob